Business domain modules – Organizing Your Application
An application will serve one or more user workflows. This type of module aims to group these flows based on the affinity of the interfaces that compose them. For example, in an application for resource management, we can have the accounting module and the inventory module.
In the application available in the ch2 folder, there is the talktalk application that we will use in this and other chapters to put our knowledge into practice. In the project folder, let’s create the home module with the following command:
ng g m home
In this command, we use the Angular CLI, ng, and the abbreviations g for generate and m for module. Then we give the name of the module, home.
Let’s create the Page component that will represent the application’s home page and, since we are using Angular material, we will use the Angular CLI to generate a page with a side menu using the following command:
ng generate @angular/material:navigation home/home
The Angular CLI, besides creating the component, also edited the home.module.ts file by adding it to the declarations attribute. Change this file as shown in the following example:
import { NgModule } from ‘@angular/core’;
import { CommonModule } from ‘@angular/common’;
import { HomeComponent } from ‘./home/home.component’;
import { LayoutModule } from ‘@angular/cdk/layout’;
import { MatToolbarModule } from ‘@angular/material/toolbar’;
import { MatButtonModule } from ‘@angular/material/button’;
import { MatSidenavModule } from ‘@angular/material/sidenav’;
import { MatIconModule } from ‘@angular/material/icon’;
import { MatListModule } from ‘@angular/material/list’;
@NgModule({
declarations: [HomeComponent],
imports: [
CommonModule,
LayoutModule,
MatToolbarModule,
MatButtonModule,
MatSidenavModule,
MatIconModule,
MatListModule,
],
exports: [HomeComponent],
})
export class HomeModule {}
In this module, we will export the HomeComponent component to use in the application’s route. In the app.module.ts file, import the module as follows:
import { NgModule } from ‘@angular/core’;
import { BrowserModule } from ‘@angular/platform-browser’;
import { AppRoutingModule } from ‘./app-routing.module’;
import { AppComponent } from ‘./app.component’;
import { BrowserAnimationsModule } from ‘@angular/platform-browser/animations’;
import { HomeModule } from ‘./home/home.module’;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
BrowserAnimationsModule,
HomeModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
With the module in the import attribute of the NgModule metadata, we can change the route in the app-routing.module.ts file:
import { NgModule } from ‘@angular/core’;
import { Routes, RouterModule } from ‘@angular/router’;
import { HomeComponent } from ‘./home/home/home.component’;
const routes: Routes = [
{ path: ”, pathMatch: ‘full’, redirectTo: ‘home’ },
{
path: ‘home’,
component: HomeComponent
},
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule],
})
export class AppRoutingModule {}
The routes component is also NgModule, however, it is specialized in organizing routes, and imports and exports only RouterModule from Angular. Here, in the routes array, we create the direction for HomeComponent.
Running the ng serve –o command, we get the application’s home page:
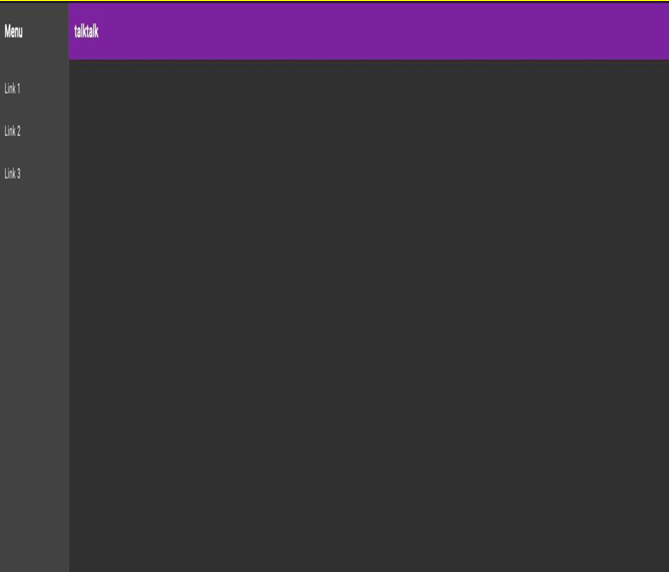
Figure 2.3 – talktalk sample application menu page