Creating methods and functions – TypeScript Patterns for Angular
One of the best ways used by TypeScript to improve the developer experience in Angular application development is through the ability to type parameters and return functions and methods.
Both for developers who create libraries and frameworks and for those who consume these software components, knowing what a function expects and what the expected return is allows us to reduce the time spent reading and looking for documentation, especially the runtime bugs that our system may encounter.
To carry out the typing of the parameters and the return of a function, let’s consider the following example:
interface invoiceItem {
product: string;
quantity: number;
price: number;
}
type Invoice = Array<invoiceItem>;
function getTotalInvoice(invoice: Invoice): number {
let invoiceTotal = invoice.reduce(
(total, item) => total + item.quantity * item.price,
0
);
return invoiceTotal;
}
export function invoiceExample() {
let example: Invoice = [
{ product: “banana”, price: 1.5, quantity: 3 },
{ product: “apple”, price: 0.5, quantity: 5 },
{ product: “pinaple”, price: 3, quantity: 12 },
];
console.log(`Invoice Total:${getTotalInvoice(example)}`);
}
In this example, we start by defining an interface that represents an invoice item and then we create a type that will represent an invoice, which in this simplification is an array of items.
This demonstrates how we can use interfaces and types to better express our TypeScript code. Soon after, we create a function that returns the total value of the invoice; as an input parameter, we receive a value with the invoice type, and the return of the function will be a number.
Finally, we create an example function to use the getTotalInvoice function. Here, in addition to type checking, if we use an editor with TypeScript support such as VS Code, we have basic documentation and autocomplete, as shown in the following screenshot:
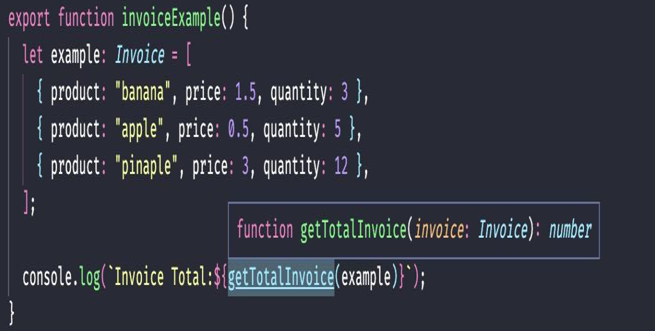
Figure 3.2 – Documentation generated by TypeScript and visualized by VS Code
In addition to primitive types and objects, functions must also be prepared to handle null data or undefined variables. In the next section, we will explore how to implement this.