Improving the size of your app – lazy loading – Organizing Your Application-2
In this file, we also removed the export of the HomeComponent component because the Home module route file will load it.
In the project’s main route file, app-routing.module.ts, let’s refactor it as follows:
import { NgModule } from ‘@angular/core’;
import { Routes, RouterModule } from ‘@angular/router’;
const routes: Routes = [
{ path: ”, pathMatch: ‘full’, redirectTo: ‘home’ },
{
path: ‘home’,
loadChildren: () =>
import(‘./home/home.module’).then((file) => file.HomeModule),
},
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule],
})
export class AppRoutingModule {}
In this code, the most important part is the loadChildren attribute. This is where we configure the lazy load, as we pass to Angular’s route mechanism a function that returns an import promise.
Note that the import function is not an Angular function, but a standard JavaScript function that allows dynamic loading of code. Angular’s route engine uses this language feature to bring this functionality.
Finally, in the main module, AppModule, let’s remove the HomeModule import:
import { NgModule } from ‘@angular/core’;
import { BrowserModule } from ‘@angular/platform-browser’;
import { AppRoutingModule } from ‘./app-routing.module’;
import { AppComponent } from ‘./app.component’;
import { BrowserAnimationsModule } from ‘@angular/platform-browser/animations’;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
BrowserAnimationsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Running our application with the ng serve command, we didn’t notice any difference. However, when executing the ng build command, we can notice the following diagnosis:
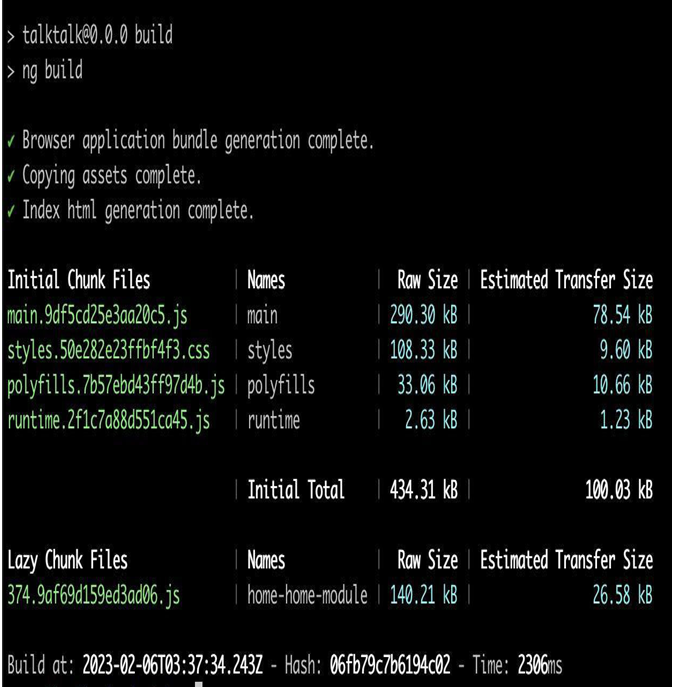
Figure 2.5 – Application bundle size after refactoring with lazy loading
The Angular build process has separated the Home module into its own bundle and the main.ts bundle has been made smaller. The difference may seem small but note that, this way, our application can scale and grow in complexity and the initial bundle will remain small or grow very little.
The new features continue to exist and be loaded by the application, but the initial loading will be faster, and these new features will be downloaded on demand only when the user accesses the route they want, giving a very positive fluidity and responsiveness.
Summary
In this chapter, we studied the Angular modules in detail and how we can use them for the organization and performance of our applications. We learned the difference between Angular modules and JavaScript modules, and we saw each attribute of a module definition and the types that we can create in the project. Finally, we learned how to avoid the single module app anti-pattern and how to create the SharedModule.
We reiterated our example application to use lazy loading of bundles, which demonstrates that good module organization reflects performance and fluidity for our users. Now, you are able to organize your application in such a way that it can scale and increase in complexity and features without compromising the maintainability of the project.
In the next chapter, we will learn how to use TypeScript effectively and productively for our Angular projects.